Angularjs MVC RESTful Web Service example
This example shows how to create a simply web application using angularjs, mvc, RESTful Web Service and ORM Entity Framework, I named it is “manage customer”.
List of features:
- List customer
- Edit customer
- Create new customer
- Delete customer
List of projects of “manage customer” application:
- CustomerEntities (Entity Framework 6.0.0.0)
- CustomerDAL (c# class library)
- CustomerBLL (c# class library)
- CustomerAPI (RESTful web service)
- CustomerUI (AngularJs, MVC)
Notes that in customer UI will be no C# controller file, it is be replaced by angularjs script file. CustomerAPI will works with CustomerBLL to controll business logic, CustomerBLL reference data layer (CustomerDAL) and CustomerDAL works CustomerEntities to query data.
OK now we focus step by step to know it.
Step 1: Create database for “manage customer” application
In this example I don’t using “code first” method so we need to create database first. This database has one “Customer” table with structure as following
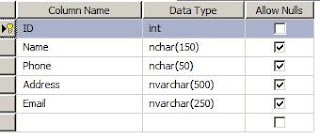
Step 2: Create CustomerEntities project using Entity Framework.
CustomerEntities be using orm Entity Framework to work with database.
On visual studio 2013 add a Class Library via name is “CustomerEntities”
Step 2.1: File=> New project => Visual c# => Class Library=>input CustomerEntities to “Name:” => OKA class library project via named “CustomerEntities” is created.
Step 2.2: Add an “Entities” folder to “CustomerEntities”, remove “Class1.cs” files
Step 2.3: Right click “Entities” => add new item=> Data => ADO.Net Enity Data Model=>Add=> EF Designer from database => Click next =>
Click OK =>
Click next
Choose tables
=> Click “Finish”
Step 2.4: Add a partial class DbContext, in this case it is “ManageCustomersEntities” class
This class is added to Entities folder via following definition
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace CustomerEntities.Entities
{
public partial class ManageCustomersEntities
{
public ManageCustomersEntities(string connectionString)
: base(connectionString)
{
}
}
}
Now project structure will be.
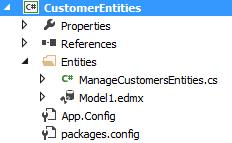
Step 3: Create CustomerDAL project
On visual studio 2013 add a ClassLibrary via name is “CustomerDAL”
Step 3.1: File=> New project => Visual c# => Class Library=>input CustomerDAL to “Name:” => OK
=>A class libaray project via named “CustomerDAL” is created.
Step 3.2: Add reference “CustomerEntities” project to this.
Step 3.3: Add “BaseDal” class to project to initial the ManageCustomersEntities.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using CustomerEntities.Entities;
using System.Configuration;
namespace CustomerDAL
{
class BaseDal
{
public ManageCustomersEntities _entities;
static string _connectionString;
public static string ConnectionString
{
get
{
if (string.IsNullOrEmpty(_connectionString) == false)
return _connectionString;
_connectionString = System.Configuration.ConfigurationManager.ConnectionStrings["ManageCustomersEntities"].ConnectionString; //
return _connectionString;
}
}
public BaseDal()
{
_entities = new ManageCustomersEntities(ConnectionString);
}
}
}
Step 3.4 Add “CustomerDal” which inherit from BaseDal
- The first, using Magane Nutget Package to install EntityFramework for CustomerDAL
- Add CustomerDal via following code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using CustomerEntities.Entities;
namespace CustomerDAL
{
class CustomerDal
{
public Customer[] GetALL()
{
ManageCustomersEntities ctx = new ManageCustomersEntities();
return ctx.Customers.ToArray();
}
public Customer GetByID(int id)
{
ManageCustomersEntities ctx = new ManageCustomersEntities();
return ctx.Customers.Where(c => c.ID == id).ToArray()[0] as Customer;
}
public bool Insert(Customer cus)
{
try
{
ManageCustomersEntities ctx = new ManageCustomersEntities();
ctx.Customers.Add(cus);
ctx.SaveChanges();
return true;
}
catch
{
return false;
}
}
public bool Update(int id, Customer cus)
{
try
{
using (ManageCustomersEntities ctx = new ManageCustomersEntities())
{
var _ob = ctx.Customers.SingleOrDefault(b => b.ID == id);
if (_ob != null)
{
_ob.Name = cus.Name.Trim();
_ob.Phone = cus.Phone.Trim();
_ob.Email = cus.Email.Trim();
_ob.Address = cus.Address.Trim();
ctx.SaveChanges();
}
}
return true;
}
catch
{
return false;
}
}
public bool Delete(int id)
{
try
{
using (ManageCustomersEntities ctx = new ManageCustomersEntities())
{
var _ob = ctx.Customers.SingleOrDefault(b => b.ID == id);
if (_ob != null)
{
ctx.Customers.Remove(_ob);
ctx.SaveChanges();
}
}
return true;
}
catch
{
return false;
}
}
}
}
Step 4: Create CustomerBLL project
On visual studio 2013 add a Class Library via name is “CustomerDAL”
Step 4.1: File=> New project => Visual c# => Class Library=> input CustomerBLL to “Name:” => OK
=> A class library project via named “CustomerBLL” is created.
Step 4.2: Add reference “CustomerEntities” and “CustomerDAL” projects to this.
Step 4.3 Add “CusBLL” class via following code
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using CustomerEntities.Entities;
using CustomerDAL;
namespace CustomerBLL
{
public class CusDLL
{
public Customer[] GetAll()
{
CustomerDal CusDAL = new CustomerDal();
return CusDAL.GetALL();
}
public Customer GetById(int id)
{
CustomerDal CusDAL = new CustomerDal();
return CusDAL.GetByID(id);
}
public void Insert(Customer cus)
{
CustomerDal CusDAL = new CustomerDal();
}
public void Update(Customer cus, int id)
{
CustomerDal CusDAL = new CustomerDal();
CusDAL.Update( id,cus);
}
public void Delete(int id)
{
CustomerDal CusDAL = new CustomerDal();
CusDAL.Delete(id);
}
}
}
The solution will be as below:
Step 5: Create CustomerAPI (RESTful web service)
On visual studio 2013 add a ClassLibrary via name is “CustomerDAL”
File=> New project => Visual c# => Class Library=> input CustomerAPI to “Name:” => OK
Step 5.1: Add references
• Add “CustomerEntities” and CustomerBLL project to this
• Add System.Web.Http and System.Net.Http
Step 5.2: Add “Controllers” folder and then add “CustomerAPIController.cs” to this folder.
Step 5.3 In “CustomerAPIController.cs” implement Get, Post, Delete RESTful webservice methods.
Code of this class as below:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Web.Http;
using System.Net.Http;
using CustomerEntities.Entities;
using CustomerBLL;
namespace CustomerAPI.Controllers
{
public class CustomerAPIController : ApiController
{
public CustomerAPIController()
{
}
[AllowAnonymous]
public Customer[] Get()
{
var cusDLL = new CusDLL();
return cusDLL.GetAll();
}
[HttpPost]
public HttpResponseMessage Post(Customer customer)
{
var cusDLL = new CusDLL();
if (customer.ID == 0)
{
cusDLL.Insert(customer);
}
else
{
cusDLL.Update( customer,customer.ID);
}
HttpResponseMessage response = Request.CreateResponse<Customer>(System.Net.HttpStatusCode.Created, customer);
return response;
}
// DELETE api/values/5
[HttpDelete]
public virtual HttpResponseMessage Delete(int id)
{
var cusDLL = new CusDLL();
if (id != 0)
{
cusDLL.Delete(id);
}
HttpResponseMessage response = Request.CreateResponse<int>(System.Net.HttpStatusCode.Created, id);
return response;
}
// GET api/values/5
public string Get(int id)
{
return "value";
}
// PUT api/values/5
[HttpPut]
public void Put(int id, [FromBody]string value)
{
}
}
}
OK now I’ve done CustomerAPI (RESTful web service), next step will UI of application.
Step 6: CustomerUI (AngularJs, MVC)
Step 6.1: File=> New project => Visual c# => ASP.Net Web Application=> input CustomerUI to “Name:” => OK
Choose Web API anf then click OK
Now solution structure will be as below:
Step 6.1: Move c# controller to CustomerAPI class library.
Our goal is no c# controller and model files in CustomerUI, so we will move all of c# controller and model files to CustomerAPI
Now have no controller and model in CustomerUI.
Step 6.2: On CustomerAPI add SetupController.cs at root
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Web.Routing;
using System.Web.Mvc;
namespace CustomerAPI
{
public class SetupController
{
public static void Setup()
{
SetupControllers();
}
private static void SetupControllers()
{
ControllerBuilder.Current.DefaultNamespaces.Add("CustomerAPI.Controllers");
}
}
}
This code will make controller builder for namespace "CustomerAPI.Controllers" which is namesapce of HomeController and ValuesController.
Step 6.3: On CustomerUI modify Global.asax.cs as following code
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Http;
using System.Web.Mvc;
using System.Web.Optimization;
using System.Web.Routing;
using CustomerAPI;
namespace CustomerUI
{
public class WebApiApplication : System.Web.HttpApplication
{
protected void Application_Start()
{
CustomerAPI.SetupController.Setup();
AreaRegistration.RegisterAllAreas();
GlobalConfiguration.Configure(WebApiConfig.Register);
FilterConfig.RegisterGlobalFilters(GlobalFilters.Filters);
RouteConfig.RegisterRoutes(RouteTable.Routes);
BundleConfig.RegisterBundles(BundleTable.Bundles);
}
}
}
Step 6.4: Run CustomerUI make sure that home page is displayed without error.
Step 7: Add angularJs and build UI for application
Now we ready to build UI for this application
Step 7.1: Add angularJs to application
Find View->Shared-> _Layout.cshtml
Add following code in <head> tag
<script src="//ajax.googleapis.com/ajax/libs/angularjs/1.3.2/angular.min.js"></script>
Step 7.2: Modify _Layout.cshtml
- Change application name
@Html.ActionLink("Application name", "Index", "Home", new { area = "" }, new { @class = "navbar-brand" })
Change to
@Html.ActionLink("Manage Customer", "Index", "Home", new { area = "" }, new { @class = "navbar-brand" })
- Add Customer Action link
<ul class="nav navbar-nav">
<li>@Html.ActionLink("Home", "Index", "Home", new { area = "" }, null)</li>
<li>@Html.ActionLink("API", "Index", "Help", new { area = "" }, null)</li>
</ul
>Change to
<ul class="nav navbar-nav">
<li>@Html.ActionLink("Home", "Index", "Home", new { area = "" }, null)</li>
<li>@Html.ActionLink("API", "Index", "Help", new { area = "" }, null)</li>
<li>@Html.ActionLink("Customers", "Index", "Customer", new { area = "" }, null)</li>
</ul>
Step 7.3: Add Customer controller for “Manage cutomer” action link
On CustomerAPI project add CustomerController.cs to Controller folder
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
namespace CustomerUI.Controllers
{
public class CustomerController : Controller
{
public ActionResult Index()
{
ViewBag.Title = "Customer Page";
return View();
}
}
}
Step 7.4: Add view for “Manage cutomer” action link
On CustomerUI add Index.cshtml “View-> Customer-> Index.cshtml”
The Index.cshtml will be contains two parts: one is list of customer and one is create or edit form, so I’ll create two html files: “list_.html “ will be list of customers and “Create_.html” will be create new or edit customer.
And the Index.cshtml code will be as below:
@{
ViewBag.Title = "Index";
Layout = "~/Views/Shared/_Layout.cshtml";
}
<br />
<br />
<div ng-app="mycustomersApp" ng-controller="customersCtrl">
<div class="container">
<div ng-include="'/Templates/list_.html'">aa</div>
<div ng-include="'/Templates/Create_.html'">aab</div>
</div>
</div>
<script src="~/AppScripts/customer.js"></script>
Note that: appscripts/customers.js will be created in next step. This script will be dedine AngularJS app as “mycustomersApp” and angularJs controller as “customersCtrl”.
Step 7.5: Add “customers.js”
On CustomerUI project create “AppScripts” folder
Add “customers.js” to this folder via below code:
var module = angular.module('mycustomersApp', []);
module.controller('customersCtrl', function ($scope, $http) {
// Get customer list
$http.get("/api/customerapi")
.success(function (response) {
$scope.Customer = response
});
// Initial
$scope.edit = true;
$scope.error = false;
$scope.incomplete = false;
// Edit
$scope.editCustomer = function (id) {
if (id == 'new') {
$scope.edit = true;
$scope.incomplete = true;
$scope.ID = 0;
$scope.Name = '';
$scope.Phone = '';
$scope.Address = '';
$scope.Email = '';
} else {
$scope.edit = false;
$scope.ID = $scope.Customer[id].ID;
$scope.Name = $scope.Customer[id].Name.trim();
$scope.Phone = $scope.Customer[id].Phone.trim();
$scope.Address = $scope.Customer[id].Address.trim();
$scope.Email = $scope.Customer[id].Email.trim();
$("#idEmail").val($scope.Email.trim());
$scope.incomplete = false;
}
};
// Update or add new one
$scope.PostCustomer = function () {
$.post("api/customerapi",
$("#cusForm").serialize(),
function (value) {
// Refresh list
$http.get("/api/customerapi")
.success(function (response) {
$scope.Customer = response
});
alert("Saved successfully.");
},
"json"
);
}
// Delete one customer based on id.
$scope.delCustomer = function (id) {
if (confirm("Are you sure you want to delete this customer?")) {
// todo code for deletion
$http.delete("api/customerapi/" + id).success(function () {
alert("Deleted successfully.");
// Refresh list
$http.get("/api/customerapi")
.success(function (response) {
$scope.Customer = response
});
}).error(function () {
alert("Error.");
});
}
};
});
This script define all actions for customer page (get list, add new, edit and delete customer)
Step 7.6: Add “list_.html” and “Create_.html'
On CustomerUI project create “Templates” folder
- Add “list_.html” to this folder via below code:
<h3>List Customers</h3>
<table class="sample-show-hide table ">
<thead>
<tr>
<th>Edit</th>
<th>Name</th>
<th>Phone</th>
<th>Address</th>
<th>Email</th>
</tr>
</thead>
<tbody>
<tr ng-repeat="c in Customer">
<td ng-class-odd="'odd'">
<button class=" btn" ng-click="editCustomer($index)">
<span class="glyphicon glyphicon-pencil" style="color:blue"></span> Edit
</button>
<button class="btn" ng-click="delCustomer(c.ID)">
<span class="glyphicon glyphicon-remove" style="color:red"></span> Delete
</button>
</td>
<td ng-class-odd="'odd'">{{ c.Name }}</td>
<td ng-class-odd="'odd'">{{ c.Phone }}</td>
<td ng-class-odd="'odd'">{{ c.Address }}</td>
<td ng-class-odd="'odd'">{{ c.Email }}</td>
</tr>
</tbody>
</table>
- Add “Create_.html” to this folder via below code:
<button class="btn btn-success" ng-click="editCustomer('new')">
<span class="glyphicon glyphicon-user"></span> Create New Customer
</button>
<hr>
<h3 ng-show="edit">Create Customer:</h3>
<h3 ng-hide="edit">Edit Customer:</h3>
<form id="cusForm" name="cusForm" class="form-horizontal" method="post" >
<input type="text" hidden="hidden" ng-model="ID" name="ID">
<div class="form-group">
<label class="col-sm-2 control-label">Name:</label>
<div class="col-sm-10">
<input type="text" ng-model="Name" placeholder="Customer name" name="Name" class="my-input" required>
{{Name}}
</div>
</div>
<div class="form-group">
<label class="col-sm-2 control-label">Phone:</label>
<div class="col-sm-10">
<input type="text" ng-model="Phone" placeholder="Phone" name="Phone" class="my-input" required>
</div>
</div>
<div class="form-group">
<label class="col-sm-2 control-label">Address:</label>
<div class="col-sm-10">
<input type="text" ng-model="Address" placeholder="Address" name="Address">
</div>
</div>
<div class="form-group">
<label class="col-sm-2 control-label">Email:</label>
<div class="col-sm-10">
<input type="email" ng-model="Email" name="Email" placeholder="Email" id="idEmail" required class="my-input">
<span class="error" ng-show="myForm.input.$error.required">
Required!
</span>
<span class="error" ng-show="myForm.input.$error.email">
Not valid email!
</span>
</div>
</div>
<hr>
</form>
<button id="saveChange" class="btn btn-success" ng-disabled="error" ng-click="PostCustomer()">
<span class="glyphicon glyphicon-save"></span> Save Changes
</button>
Step 7.7: Link CustomerAPI to CustomerUI
Because Controller ang ControllerAPI move CustomerAPI project, so to in this step we will answer the question “How CustomerUI use CustomerAPI as its controller?”. Please follow steps
- Add CustomAssemblyResolver.cs in App_Start via below code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Http.Dispatcher;
using System.Reflection;
using CustomerAPI.Controllers;
namespace CustomerUI.App_Start
{
public class CustomAssemblyResolver : IAssembliesResolver
{
public ICollection<Assembly> GetAssemblies()
{
List<Assembly> baseAssemblies = AppDomain.CurrentDomain.GetAssemblies().ToList();
var controllersAssembly = Assembly.LoadFrom(@"CustomerAPI.dll");
baseAssemblies.Add(controllersAssembly);
return baseAssemblies;
}
}
}
- Modify Global.asax.cs by add
GlobalConfiguration.Configuration.Services.Replace(typeof(IAssembliesResolver), new CustomAssemblyResolver());
Step 7.8: Add more CSS for “list_.html” and “Create_.html'.
Add “CustomerPage.css” file to “Content” folder of CustomerUI project
td {
padding-right: 30px;
}
.red {
padding: 20px;
background-color: red;
color: #FFF;
}
.border {
border: solid 1px blue;
}
.base-class {
-webkit-transition: all cubic-bezier(0.250, 0.460, 0.450, 0.940) 0.5s;
transition: all cubic-bezier(0.250, 0.460, 0.450, 0.940) 0.5s;
}
.base-class.my-class {
color: red;
font-size: 3em;
}
.odd {
color: #0094ff;
}
.rowEvent {
color: #808080;
}
.my-input {
-webkit-transition: all linear 0.5s;
transition: all linear 0.5s;
background: transparent;
}
.my-input.ng-invalid {
color: #a50e0e;
background: #6f041e;
}
.sample-show-hide {
-webkit-transition: all linear 0.5s;
transition: all linear 0.5s;
background: transparent;
}
- Modify View\Shared\_Layout.cshtml by add following code to <Head> tag.
<link href="~/Content/CustomerPage.css" rel="stylesheet" />
Step 7.9: Add connection string to web.config of CustomerUI.
Open App.confg of CustomerEntities, copy the “connectionstrings “tag paste override exist “Connectionstirngs” of CustomerUI.
It will be like below:
<connectionStrings>
<add name="ManageCustomersEntities" connectionString="metadata=res://*/Entities.Model1.csdl|res://*/Entities.Model1.ssdl|res://*/Entities.Model1.msl;provider=System.Data.SqlClient;provider connection string="data source=EZITWK106\LOCALSQL2008R2;initial catalog=ManageCustomers;persist security info=True;user id=sa;password=855554;MultipleActiveResultSets=True;App=EntityFramework"" providerName="System.Data.EntityClient" />
</connectionStrings>
Step 7.10: security notes.
At this application I don’t care about security for RESTFul webservice, encrypt and decrypt connection string… you can do it more to your application.
Done – Following is screen shot of Customer page.
thanks man !
ReplyDeletefinally a "real" world example that use "real" world scenario to explain things !!!!
does exist a similar concept like in WPF for observablecollections and databinding in angularJs ? if so i would love to read an article about that .
again, a real world example with database would be 10X more usefull, for example an invoice list example with databiding to the list, counts, summaries and things like that would be just perfect .
thanka again .
if you have any pagination for MVC with AngularJs sample format. Please let me know.
ReplyDeleteYou can refer to http://angularjsaz.blogspot.com/2015/09/angularjs-table-sorting-filter-and.html . It is basic but you can customize it to use.
ReplyDeleteI dont know what is the hyper with Angular. I can do all this tutorial only with jquery. Can somebody explain me??
ReplyDelete@Chocobomix Yes you can easily do this with jquery and there is nothing wrong in it but let say if you want to write unit test or test your javascript code in isolation then can you do it? AngularJS gives you the notion of dependency injection so that you build your services and can swap them as you need. This way you can test your AngularJS code without connecting to Web API or any external dependencies.If testing is not your thing then you can do with AngularJS, also there are some nice concepts like directives,Services etc which makes programming fun so hence some people love AngularJS :)
ReplyDeletevery poor job. the code is incomplete and full of bugs. what is the reason to publish nonworking code? have you ever test your solution?
ReplyDeleteThis might help also, Check this one out.
ReplyDeletehttps://www.ziptask.com/AngularJS-When-JavaScript-Met-MVC
This comment has been removed by a blog administrator.
ReplyDeleteAgree Al Bundy
ReplyDeleteRecently started learning AngularJS. Very well explained !!
ReplyDeletethanks for sharing. for more #angularjs #mvc article visit #aspmantra
ReplyDeleteI really appreciate information shared above. It’s of great help. If someone want to learn Online (Virtual) instructor lead live training in ANGULAR JS, kindly contact us http://www.maxmunus.com/contact
ReplyDeleteMaxMunus Offer World Class Virtual Instructor led training on ANGULAR JS. We have industry expert trainer. We provide Training Material and Software Support. MaxMunus has successfully conducted 100000+ trainings in India, USA, UK, Australlia, Switzerland, Qatar, Saudi Arabia, Bangladesh, Bahrain and UAE etc.
For Demo Contact us.
Nitesh Kumar
MaxMunus
E-mail: nitesh@maxmunus.com
Skype id: nitesh_maxmunus
Ph:(+91) 8553912023
http://www.maxmunus.com/
Those are some great tips!! I will definitely have to remember those!
ReplyDeletehire angularjs developer
By the way, if you want to hire AngularJS developer, there are a lot of things you should know: developer's skills, roles and responsibilities, what hard skills and soft skills questions you should ask them, where you can find web developers and what is hourly rate of Angular dev in various regions of the world. Check this article to learn more about it.
Deleteangular web API working with PHP and ASP.net
ReplyDeleteregistration and login form in php and mysql with validation code free download
codeigniter login and registration
angular 4 login page example
admin panel in php
angular 4 login example
angularjs datatable demo
angular 4 simple login example
SEO needs to be implemented in a way that is effective in achieving your SEO goals and providing that all important meaningful presence on the World Wide Web.Blog Comment
ReplyDeleteWOW Awesome Information, I like your Post. Thanks for Information.
ReplyDeleteDigital Marketing Company SEO Services Company
Website Designing Company
Your article is very interesting. Your level of thinking is good and the clarity of writing is excellent. I enjoyed so much to read this blog. seo services in kolkata | seo company in kolkata | seo service provider in kolkata | seo companies in kolkata | seo expert in kolkata | seo service in kolkata | seo company in india | best seo services in kolkata | digital marketing company in kolkata | website design company in kolkata
ReplyDeleteIts good acritical to learn angular...
ReplyDeleteAngular JS Online training
Angular JS training in hyderabad
Post is informative & helpful.
ReplyDeleteseo company in Bangalore
these are the study abroad consultants in Kerala where aspiring students gets unlimited range of scope to study abroad.
ReplyDeleteStudy abroad Consultants in Kerala
ReplyDeleteIt's Really A Great Post. Looking For Some More Stuff.
Digital marketing agency in hyderabad | Digital marketing companies in hyderabad
SIAUAE is one of the top audit firms in UAE with highly experienced professionals. As a leading Audit Firms in UAE , we achieved the label of best tax consultant in UAE.Our CA services Will help you.
ReplyDeleteWe providing the best VAT consultancy services in UAE .As a leading VAT Consultants in UAE , we achieved the label of best VAT consultant in UAE.
Looking for the top accounting firms in UAE? SIAUAE is the best Accounting Firms in UAE , we offer comprehensive professional services in UAE.
SIAUAE management consultancy is a professional Management Consultancy UAE , Helping clients achieve their business goals. For more details call us now.
Good Blog, thanks for sharing
ReplyDeletethe intensity of smells can't be exaggerated. Smell has such a solid association with one's passionate express that it revives the recollections that went with our experience, in a few occasions, returning over numerous years. A deliberately built fragrance can lift one's state of mind, and places one out of a positive and innovative outlook. Our fragrance arrangements give you a chance to make the ideal experience for your customers and associates – one in which they remain drew in and associated.
Aroma diffuser
Good Blog, thanks for sharing
ReplyDeleteToday the companies are continually being challenged to reduce the expenses, most of working expenditures and other requirements. The main costs include the salary of employees like HR professional, accountant, auditors and administration. These problem can be overcome by best expert business outsourcing company. They provide quality and valuable services at low cost for the business enterprises. In UAE most of accounts payable outsourcing companies offers cost effective accounting services that will help the companies effectively in the current situation.
Audit firms in UAE
ISO Certification in Delhi – Genveritas a global ISO Certification Consulting firm represents considerable authority in tweaked and result-situated answers for assisting organizations to actualize change and improve business execution.
ReplyDeleteNeuro Doctors are a cohesive group of Top Neurosurgeon in Bangalore Neurologists, Intervention Neuroradiologist, pain management specialists who work together to provide comprehensive neurosciences care to our patients.
KEEN SEO Agency – Best Web Design Company in Bangalore . We provide full-service Web Design & Development Solutions that also includes specialized SEO services for Small Businesses. We offer Strategist Local SEO, Ecommerce SEO, website auditing, Paid Search (PPC) strategies including Google Ads, Facebook & Linked In Ads for Small Business (B2B & B2C).
This comment has been removed by the author.
ReplyDeleteI really enjoyed reading this blog. It was explained and structured with perfection; Best Digital Marketing Company in Delhi
ReplyDeleteThanks For Sharing The Information The Information Shared Is Very Valuable Please Keep Updating Us Time Just Went On Reading The article
ReplyDeleteBy Cloudi5 is the Web Design Company in Coimbatore
great blog. Really love your content do check Agricultural Investment Company
ReplyDeleteBest Agriculture Financial Company in India
I really appreciate your articles im a daily reader of your articles i love your informative tips and paragraph which are rich of useful knowledge thanks for sharing.
ReplyDeleteCheck Out The Best Digital Marketing Company in Bangalore|
Top igital Marketing Companies In Bangalore|
Digital Marketing Companies in Bangalore|
Digital Marketing Services In Bangalore|
Best Digital Marketing Company In Bangalore|
Gladias guarantees that the highly qualified and trained Auditors are assigned to work with you will be the same through-out the process in order to sustain continuity.
ReplyDeletehttps://www.gladiasconsulting.com/iso/
Thanks for sharing this wonderful content. it's very useful to us. I gained a lot of information, the way you have clearly explained is really fantastic. Thanks a lot for this blog.
ReplyDeleteHire Dedicated Angularjs Developers
Nice post really useful information. We are the leading website development services in dubai. Hire our web design agency in dubai today for web design services in dubai
ReplyDeletehttps://imaniaci.blogspot.com/2015/05/la-google-car-debutta-su-strada.html?showComment=1626433675825#c3147092962344033385
ReplyDeleteNice post it is really an interesting article.We are also providing the web design services in mumbai. We are the leading
ReplyDeleteweb design companies in mumbai
website designers in mumbai
Great Article it its really informative and innovative keep us posted with new updates. it was really valuable. thanks a lot.
ReplyDeleteISO 9001 Certification in qatar
Hi thanks for sharing the blog and it has a useful information
ReplyDeleteSTARTUP COMPANY REGISTRATION in Bangalore
Hi thanks for sharing this blog and it is informative
ReplyDeleteSTARTUP COMPANY REGISTRATION in Bangalore
Our company is a one-stop solution for all your needs related to web designing. We have been in this business from past many years and are one of the web designing company in Chandigarh . In the times, where website is an essential requirement for your business, you should not settle for anything but the best. We specialize in PHP website development, Magento website development, website optimization, WordPress website development and custom website designing. We cater to the fraternity of clients and provide them websites according to their business needs and our websites have been loved by the Aspiring businesses, Emerging industry leaders and quality driven clients hire us to build effective websites for them to engage in digital world in Chandigarh and all across the world.
ReplyDeleteweb development company in Chandigarh
Hi,i would like to tell is it is a perfect platform acquire my knowledge of skillset needed to me,and good blog as well.
ReplyDeleteBest Web designing company in Hyderabad
really liked this content, loved reading & viewing.
ReplyDeleteSearch engine optimization agency
hello very nice blog. it is really an interesting article we are providing some backlinks. I hope these content useful for you.
ReplyDeletebacklinks
backlinks
backlinks
backlinks
backlinks
backlinks
backlinks
backlinks
https://www.certvalue.com/iso-14001-certification-in-bahrain/
ReplyDeleteCertvalue is the top ISO 14001 Consultants in Bahrain for providing ISO 14001 Certification in Manama, Riffa, Hamad Town, Sitra ,Budaiya and other major Cities in Bahrain with services of implementation.
I am very proud to study such an informative blog. i Will observe your updates in future so, please add greater and extra ideas.
ReplyDeleteBacklinks
Backlinks
Backlinks
Backlinks
Hi thanks for sharing this do follow backlinks it was so useful
ReplyDeleteDo Backlinks
Do Backlinks
Do Backlinks
Nice post it is really an interesting article. We are also providing the ISO 27001 certification services in Qatar. We are the leading
ReplyDeletebacklinks
backlinks
backlinks
backlinks
backlinks
backlinks
backlinks
backlinks
Hi thanks for sharing amazing do follow backlinks
ReplyDeleteDo Backlinks
Do Backlinks
Do Backlinks
Hello everyone These are the top Digital Marketing organizing organizations in Dubai business viability with effective development and arrangement.
ReplyDeleteBacklinks
Backlinks
As one of the leading Web designing company in Mohali, we cater to the fraternity of the clients and provide them the services which suits best to their requirements. We analyze the situation of every business deeply, understand their purpose and comprehend with it accordingly. Our services include web designing, Logo Design, web development and search engine optimization. We provide websites to our clients which are very cost efficiently. We work in all the industries from fashion to Entertainment to health care and real estate. When it comes to your business you should not take chances but rather go for the best and we at PPC Fame provide you with the best resolutions. We are the first preference for the industry leaders and well settled businesses when it comes to best Web designing company in Mohali.
ReplyDeleteWeb designing company in Mohali
Nextwave Creators is not to be missed if the topic is the best PPC Company in Bangalore. They view your website through their team of experts who have digital marketing consultants and online marketing analysts. You can lead the company for a limited period of time.PPC Company in Bangalore
ReplyDeleteHello Everyone It is nice post and I found some interesting information on this blog, keep it up. Thanks for sharing.
ReplyDeletebacklinks
backlinks
backlinks
backlinks
ReplyDeleteThis post will be very useful to us....i like your blog and helpful to me..
Hire Remote Angularjs Developer in India
Hello Everyone! Great article! This is very necessary archives for us. I like all content material fabric and information. I have observe it. You recognize more about this please go to againbacklinks
ReplyDeletebacklinks
backlinks
backlinks
backlinks
Digital Marketing Institute in Panchkula
ReplyDeleteGreat blog.thanks for sharing such a useful information
ReplyDeletebest seo company in bangalore
best seo services company in bangalore
Thanks for Sharing Great post
ReplyDeleteBuy Plants Online in India
Buy Seeds Online in India
Buy Handicrafts Online
Buy Plant Care Products
Buy Home Decor Items Online India
Buy Planter Pot & Flower Stands
Buy
Succulents & Cactus
Exellent your post. i reaching daily on your blogs. thanks for sharing this informative information.
ReplyDeleteBuy YouTube Views
Buy YouTube Subscribers
Buy YouTube Live Stream Views
Facebook Live Views
Free Instagram Reels Views
Instagram Live Views
Instagram Story Views
Facebook Views
Grocery Unto 45% Off on Online Grocery Shopping-CapitalGrocery.in
ReplyDeleteSaffola Gold Pro Healthy Lifestyle Edible Oil (Pouch) (1 litre)
Saffola Gold Pro Healthy Lifestyle Edible Oil (Jar) (2 litre)
Saffola Active Pro Weight Watchers Edible Oil (Pouch) (1 litre)
Fortune Xpert Pro Sugar Conscious Edible Oil (Jar) (5 litre)
Fortune Xpert Pro Sugar Conscious Edible Oil (Pouch) (1 litre)
Thanks for sharing the valuable information
ReplyDeleteHire Angularjs Development company in India
Thanks for sharing
ReplyDeleteVillage Talkies a top-quality professional corporate video production company in Bangalore and also best explainer video company in Bangalore & animation video makers in Bangalore, Chennai, India & Maryland, Baltimore, USA provides Corporate & Brand films, Promotional, Marketing videos & Training videos, Product demo videos, Employee videos, Product video explainers, eLearning videos, 2d Animation, 3d Animation, Motion Graphics, Whiteboard Explainer videos Client Testimonial Videos, Video Presentation and more for all start-ups, industries, and corporate companies. From scripting to corporate video production services, explainer & 3d, 2d animation video production , our solutions are customized to your budget, timeline, and to meet the company goals and objectives.
As a best video production company in Bangalore, we produce quality and creative videos to our clients.
Well explained! Here, I've a researched piece of article drawing line between two famous front-end development frameworks; Angular and React .
ReplyDeleteBusiness Management Assignment Help
ReplyDeleteWe help students to pursue higher studies (UG and PG courses) abroad and look after their admission procedures. Proper Visa counselling and all support services are provided under one name, Sign Overseas. Students choose us, because we find the right institution for them abroad, according to their preferences. We keep in mind the financial, educational, and personal constraints.
ReplyDeletesignoverseasedu.com
Custom website applications will save lots of time and money for your business. Think of the activities that take the most time and how awesome it will be to automate them! Your conversion rates can also be multiplied by a custom web app, because these apps are designed to get the highest possible user engagement.
Our subject matter experts have extensive knowledge and experience in a wide range of fields, and they consistently provide outstanding results. They provide professional Business Management Assignment Help as well as online business management assignment help. They could be able to assist students with research articles and writings in business.
ReplyDeleteThis comment has been removed by the author.
ReplyDeleteAmazing article. It's very useful.
ReplyDeleteIt looks like you have put lot of work into this.
SMARS designs jewelry to run along with your ever-changing wardrobe. A piece of Jewelry can either make or break your entire look; therefore, every unique outfit needs a different piece of jewelry to compliment it. But looking at the prices of traditional jewelry, we usually find occasions like festivals or ceremonies to buy it. And these adorable pieces spend most of their lives in the lockers. Komal, the founder of SMARS, understood this gap in the market. Every single piece is limited edition and walks hand-in-hand with trends. Adored by customers from all over the world, we ensure the quality delivery of our high-end, Indian fashion costume jewelry. Shop online for latest collection of Kundan, antique and temple jewelry in India check out necklace sets, earrings, bangles, chokers for girls and many more Indian jewelry sets for women available with free shipping across India.
Take a look: Buy Chokers For Womens Online
A Professional ISO consultancy company in Coimbatore - ENN Consultancy. We provide iso 9001, iso 22000, iso 14001 consultants Services, and Training in Salem, Erode, Tirupur, Trichy, Madurai, Karur, and Chennai.
ReplyDeleteVisit EnnConsultancy
An interesting and amazing post Really helpful . Thank You for your sharing.
ReplyDeleteStudy Abroad Consultants in Kochi
Nice post. I found it very useful. Immigration Consultants in Kochi
ReplyDeleteThanks for Sharing. We are well-known as a Social Media Marketing Company in Dubai. We provide quality services and advertising and lead generation
ReplyDeleteYour blog is really very nice. I came to know many useful information.Study Abroad Consultants in Kochi
ReplyDeleteCanApprove Immigration Servicesis the most trusted name in the immigration consultancy services sector in Thiruvalla. The largest town in the district of Pathanamthitta in Kerala, Thiruvalla is known as the land of non-resident Indians. The town is home to people who have proved that they can dream big and have the potential to make it big in any part of the world.
ReplyDeleteVery nicely explain each and every point of this blog. I must say you have done good research before writing the blog. There are some students who faced so many problem writing their own assignments. If you are one of them. then, solution is "BookMyEssay". We are well known organization that gains popularity by delivering Research Writing Help and 100% plagiarism free content.
ReplyDeleteThanks for sharing. Bedigitech presenting SEO Services in India. As a result, our clients have achieved good search engine rankings with high-quality traffic.
ReplyDeleteThis comment has been removed by the author.
ReplyDeleteThanks for the information shared. Keep updating blogs regularly. If you have time visit
ReplyDeletehttps://www.ennconsultancy.com/ce-marking.php
Nettoyage de maisons et appartements Lazanne
ReplyDeletehttps://nettoyage-appartements.ch/
Notre offre, comprenant le nettoyage d’appartements et de maisons à Lausanne, s’adresse aux clients qui recherchent des services professionnels, y compris des travaux de nettoyage complets. Nous les mettons en œuvre à la fois sporadiquement, sous une forme ponctuelle et périodiquement. Nous nous distinguons par l’expérience et le professionnalisme, qui s’expriment dans la plus haute qualité de services.
Реєстрація ТОВ в Києві в 2023 році від нашої компанії – це професійні послуги реєстрації товариства з обмеженою відповідальністю під ключ. Скориставшись нашими послугами і доручивши реєстрацію ТОВ нашим кваліфікованим фахівцям, які в найкоротші терміни проведуть всю необхідну роботу з оформлення паперів і спілкуванням з держслужбовцями, Вам не буде потрібно стояти в чергах і витрачати свій час на підготовку документів.
ReplyDeleteМи допомагаємо підібрати юридичну адресу в Києві і Києвській області!
https://xn----7sbcickxnhd6alkoeinny1mti.xn--j1amh/uk/reyestraciya-tov/
Коротка інформація яка необхідна, щоб зареєструвати ТОВ в Києві:
Середній термін реєстрації ТОВ займає 2 робочих дні.
Freight Forwarding Solutions is a dependable shipping partner for businesses of any size. We pride ourselves on keeping companies moving. Communication is one of our most important company values. Our freight forwarding service keeps your company informed on the status of your freight. We’re partnered with some of the best companies in the industry to provide efficient cargo transportation. International air freight is the best option for those who need fast shipping. Our air freight team meets the highest, most up-to-date standards for freight forwarding. We also offer international ocean freight forwarding, which is cost-effective and reliable. Trucking is ideal for transporting goods domestically, and we work with trusted, well-screened drivers. Our warehouse service is ideal for those who need storage for their cargo. Our climate-controlled warehouses will keep even the most delicate goods in excellent condition. Once you’re ready to ship, you can rely on our distribution service to send packages out in a timely manner. We pride ourselves on helping clients achieve their shipping goals at a reasonable rate with excellent service. For more information, contact our team in Lynbrook, NY! We’ll provide a custom quote for your shipping requests.
ReplyDeletehttps://adminfreightforwardingsolutions.com/
Nirvana Aesthetics And Wellness
ReplyDeleteSkin Rejuvenation- This minimally invasive treatment uses light to deliver significantly dramatic results that will leave your skin glowing.
HOW IT WORKS:Using light technology this procedure induces cell renewal of the dermal and epidermal skin layers to prompt the body to shed the dull, aged appearance
https://nirvanaaestheticsandwellness.org/
These are external parasites known as ticks and fleas. They can transfer several diseases in your dogs, apart from causing itching and scratching.click on k9nerds
ReplyDeleteWonderful information. I really enjoyed reading the article.
ReplyDeleteDigital Agency in London
Thanks for Sharing. "People Also Search For" is a concept created by Google in favor of putting on suggestions linked to search queries made by the user with signatures of intent. It is useful for businesses because it helps discover keywords based on relevant searches and search patterns and refine one's SEO strategy further. PASF insights are useful in optimizing content, improving search ranking, and increasing organic traffic to the website, as they show user-driven interests when searching for something.
ReplyDeleteChauffeur driver jobs offer a rewarding career with flexible schedules and competitive pay. Many companies seek experienced, professional drivers for corporate clients, luxury travel, and event transportation. Search online job portals, local agencies, or private firms to find opportunities near you and start your journey in premium transportation.
ReplyDelete